Understanding C# Polymorphism: A Comprehensive Guide
Written on
Chapter 1: Introduction to Polymorphism
Polymorphism, which translates to "many forms," is a fundamental concept in programming. It is typically realized through two main techniques: static binding and dynamic binding. Static polymorphism determines the method to execute at compile time, while dynamic polymorphism resolves this at runtime.
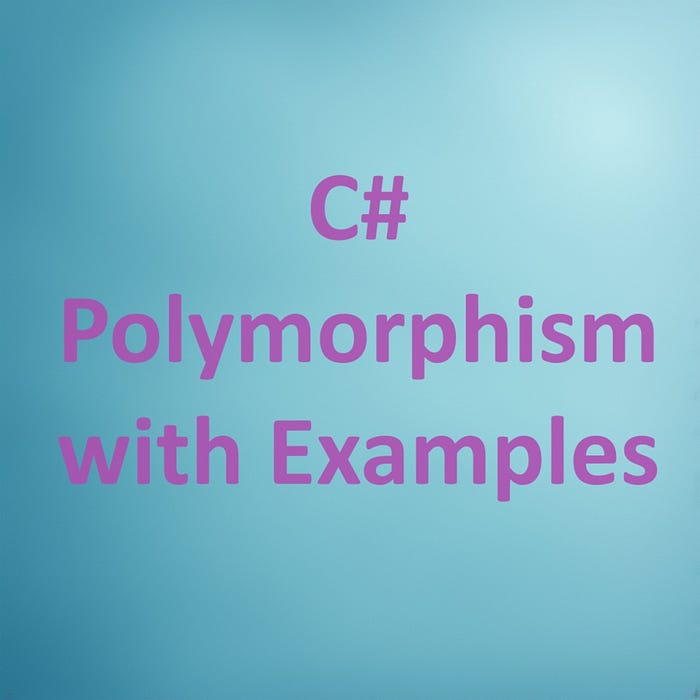
Chapter 2: Types of Polymorphism
Polymorphism in C# can be categorized into two types:
Section 2.1: Compile-Time Polymorphism (Static Binding)
Compile-time polymorphism occurs when the compiler decides which method or operator to execute based on the number and types of arguments or operands. This typically manifests through method overloading or operator overloading.
Method Overloading
Method overloading is when multiple methods in a class share the same name but differ in their parameter lists. These methods must vary in the number, type, or order of parameters.
public class FruitUtility
{
// Method with no parameters
public int AddFruitPrice()
{
return 0;}
// Method with two integer parameters
public int AddFruitPrice(int price1, int price2)
{
return price1 + price2;}
// Method with three double parameters
public double AddFruitPrice(double price1, double price2, double price3)
{
return price1 + price2 + price3;}
// Method with a different parameter type
public string AddFruitPrice(string a, string b)
{
return a + b;}
}
Section 2.2: Run-Time Polymorphism (Dynamic Binding)
Run-time polymorphism is resolved during execution and arises through method overriding. This occurs when a derived class implements methods from its base class. In the base class, the virtual keyword is used to define the method, while the override keyword is employed in the derived class to implement the base class method.
Method Overriding
When a derived class implements base class methods, it utilizes the virtual keyword in the base class and the override keyword in the derived class.
// Base class (parent class)
public class Fruit
{
public virtual void Eat()
{
Console.WriteLine("Fruit is for eating.");}
public virtual void Taste()
{
Console.WriteLine("Fruit has taste.");}
public virtual void Color()
{
Console.WriteLine("Fruit has color.");}
}
// Derived class (child class)
public class WaterMelon : Fruit
{
public override void Eat()
{
Console.WriteLine("WaterMelon is for eating.");}
public override void Taste()
{
Console.WriteLine("WaterMelon has a mixture of sweet, sour, and bitter taste.");}
public override void Color()
{
Console.WriteLine("Fruit has a dark green color.");}
}
// Derived class (child class)
public class Mango : Fruit
{
public override void Eat()
{
Console.WriteLine("Mango is for eating.");}
public override void Taste()
{
Console.WriteLine("Mango has a sweet and creamy taste.");}
public override void Color()
{
Console.WriteLine("Mango has a yellow color.");}
}
Chapter 3: Complete Example of C# Polymorphism
To illustrate both types of polymorphism, consider the following complete example:
using System;
public class Program
{
public static void Main(string[] args)
{
Fruit objFruit;
objFruit = new Fruit();
objFruit.Eat();
objFruit.Taste();
objFruit.Color();
objFruit = new WaterMelon();
objFruit.Eat();
objFruit.Taste();
objFruit.Color();
objFruit = new Mango();
objFruit.Eat();
objFruit.Taste();
objFruit.Color();
}
}
The output will be as follows:
Fruit is for eating.
Fruit has taste.
Fruit has color.
WaterMelon is for eating.
WaterMelon has a mixture of sweet, sour, and bitter taste.
Fruit has a dark green color.
Mango is for eating.
Mango has a sweet and creamy taste.
Mango has a yellow color.
Summary
In summary, compile-time polymorphism is achieved through method overloading, while run-time polymorphism is attained via method overriding. These concepts exemplify the versatility inherent in object-oriented programming.
For further enriching tutorials, please visit C-Sharp Tutorial. If you found this article helpful, consider showing appreciation by following the author.
Chapter 4: Additional Resources
The video titled "C Programming Tutorial for Beginners" provides an excellent foundation for understanding programming concepts, including polymorphism.