Understanding Text Similarity in Natural Language Processing
Written on
Chapter 1: Introduction to Text Similarity
Text similarity is a fundamental concept in Natural Language Processing (NLP). It refers to the process of assessing how closely two documents relate to each other in terms of meaning and wording.
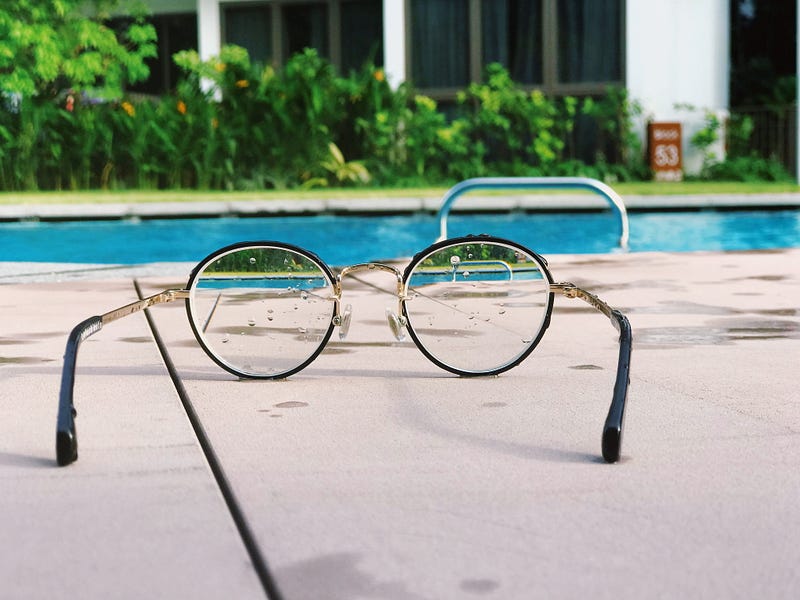
Consider these two statements:
- My house is empty.
- There is nobody at mine.
Despite their different phrasing, a human reader would recognize that both sentences convey a similar idea. The only common word is "is," which doesn't reflect their actual similarity. Thus, an effective similarity algorithm should yield a high similarity score for these sentences.
This concept is known as semantic text similarity, where the focus is on the contextual meaning of the documents. Achieving this is challenging due to the inherent complexities of natural language.
In contrast, lexical text similarity examines how similar documents are at the word level. Traditional methods often emphasize lexical similarity and tend to be quicker to implement compared to the more sophisticated deep learning approaches that have become popular recently.
In summary, text similarity can be understood as the endeavor to evaluate how "close" two documents are in terms of both lexical and semantic similarity. This is a prevalent challenge in the NLP field, with applications including assessing document relevance in search engines and recognizing similar queries to provide consistent responses in AI systems.
Section 1.1: Key Evaluation Metrics
When carrying out NLP tasks, it's vital to have metrics that help assess the quality of the work. Phrases like "the documents are similar" are subjective, whereas metrics like "the model has a 90% accuracy score" provide concrete feedback.
Common evaluation metrics for text similarity include:
- Euclidean Distance
- Cosine Similarity
- Jaccard Similarity
I discussed Euclidean Distance and Cosine Similarity in previous works, while Sanket Gupta’s article offers a thorough overview of the Jaccard similarity metric.
To illustrate these evaluation methods, let’s look at a coding example in Python.
Subsection 1.1.1: Lexical Text Similarity Example in Python
# Importing necessary libraries
import numpy as np
from sklearn.metrics.pairwise import cosine_similarity
from sklearn.feature_extraction.text import TfidfVectorizer
# Function to calculate Jaccard similarity
def jaccard_similarity(doc_1, doc_2):
a = set(doc_1.split())
b = set(doc_2.split())
c = a.intersection(b)
return float(len(c)) / (len(a) + len(b) - len(c))
# Defining the corpus
corpus = ["my house is empty", "there is no one at mine"]
# Evaluating cosine similarities with vector representations
tfidf = TfidfVectorizer()
X = tfidf.fit_transform(corpus)
# Printing results
print(f"Cosine Similarity: {cosine_similarity(X, X)[0][1]}nJaccard Similarity: {jaccard_similarity(corpus[0], corpus[1])}")
The output reveals that despite our intuition suggesting these sentences are similar, both metrics indicate they are not closely related.
Chapter 2: Semantic Text Similarity Example in Python
To analyze semantic similarity, we can use the following Python example:
from gensim import corpora
import gensim.downloader as api
from gensim.utils import simple_preprocess
from gensim.matutils import softcossim
corpus = ["my house is empty", "there is no one at mine"]
dictionary = corpora.Dictionary([simple_preprocess(doc) for doc in corpus])
glove = api.load("glove-wiki-gigaword-50")
sim_matrix = glove.similarity_matrix(dictionary=dictionary)
sent_1 = dictionary.doc2bow(simple_preprocess(corpus[0]))
sent_2 = dictionary.doc2bow(simple_preprocess(corpus[1]))
print(f"Soft Cosine Similarity: {softcossim(sent_1, sent_2, sim_matrix)}")
In this case, we find that considering the context allows us to conclude that the sentences are indeed quite similar, despite having few shared words.
The first video, "Introduction to Document Similarity," dives deeper into this topic, providing foundational insights and practical applications.
The second video, "Calculating Text Similarity in Python with NLP," offers a hands-on approach to implementing these concepts in Python.
Wrap Up
By now, you should have a clearer understanding of text similarity and the methods to evaluate it, including lexical and semantic approaches. For those seeking a career in NLP, consider developing a resume parser that assesses how closely your resume matches a job description.
Thank you for reading! Connect with me on LinkedIn and Twitter to stay informed about my latest posts on AI, Data Science, and Freelancing.