Understanding Python's Garbage Collector for Optimal Memory Management
Written on
Chapter 1: Introduction to Python's Garbage Collector
Gaining insight into Python's garbage collector is essential for developing efficient programs. In Python, all objects are stored in heap memory, which is managed privately by the interpreter. Unlike languages such as C++ or C#, Python features an automated garbage collection system that takes care of memory allocation and deallocation without requiring programmer intervention.
This article will explain the workings of the Python garbage collector.
Python's garbage collector is tasked with automatically reclaiming memory that is no longer in use. Its design prioritizes transparency and automation, alleviating programmers from the burden of manual memory management. This automatic system helps prevent memory leaks and enhances program efficiency by addressing potential memory-related issues in dynamic memory allocation.
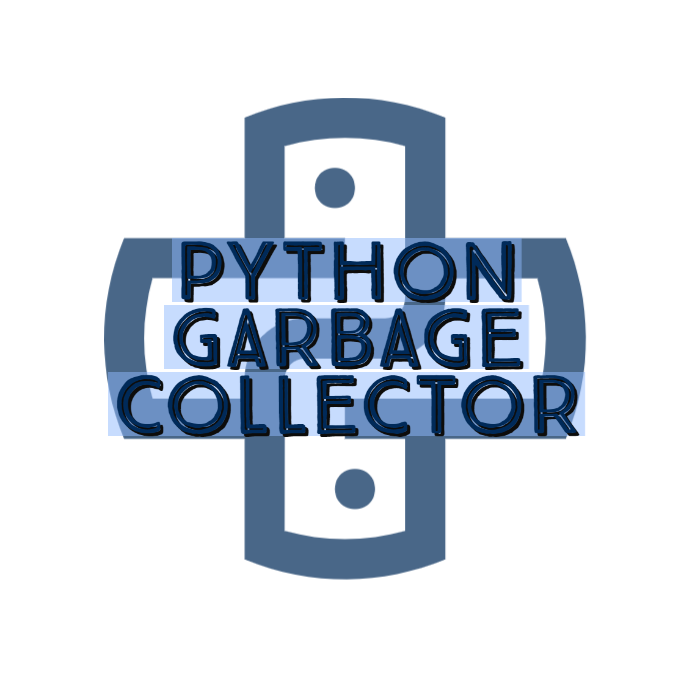
Chapter 1.1: Understanding Reference Counting
The garbage collector operates using a method known as reference counting, which tracks objects currently in use. Each time an object is created, it is assigned a reference count, representing how many references to the object exist within the program.
The garbage collector routinely conducts cycles to identify and remove objects with a reference count of zero. When an object's references are exhausted, it can be safely deleted from memory. Additionally, the garbage collector is capable of detecting cycles, such as self-referencing data structures, ensuring that all objects are appropriately deallocated.
Chapter 1.2: Cycle Detection in Garbage Collection
The cycle detection algorithm begins with a set of root objects actively utilized by the program. The garbage collector traces all references from these roots to other objects, constructing a graph of accessible objects. Any objects not reachable from this root set are classified as garbage and can be removed safely.
In scenarios where numerous objects are frequently created, manual memory management may be necessary for optimal performance.
Chapter 2: Generational Garbage Collection
Python's garbage collector employs a generational approach, consisting of three distinct generations. The cleaning process occurs periodically based on the generation to which an object belongs. Objects that remain referenced for extended periods advance to older generations.
Each generation has a specific threshold that indicates the maximum number of objects it can contain. When this threshold is exceeded, the garbage collector initiates a collection process.
Python also includes a gc module, which provides developers with tools to interface with the garbage collector. This module allows for the adjustment of collection frequency and even the option to disable the garbage collector. For example, the threshold for each generation can be customized using the gc.set_threshold function, and garbage collection can be triggered manually with gc.collect, allowing the optional specification of a generation number.
Chapter 3: Conclusion
Understanding the Python garbage collector is crucial for writing efficient code. Through reference counting and cycle detection, it effectively manages memory allocation and deallocation, promoting optimal performance in Python applications.
Discover how to adjust Python GC settings for improved performance.
An overview of garbage collection in Python and its significance.