Mastering Route Creation in React Applications
Written on
Chapter 1: The Importance of Routing in React
Implementing multiple URL routes can greatly improve the user experience of your application. Not only do routes contribute to a better site structure, but they also simplify navigation for users by directing them to specific subpages. In this article, we will explore how to effectively manage routes within a React application.
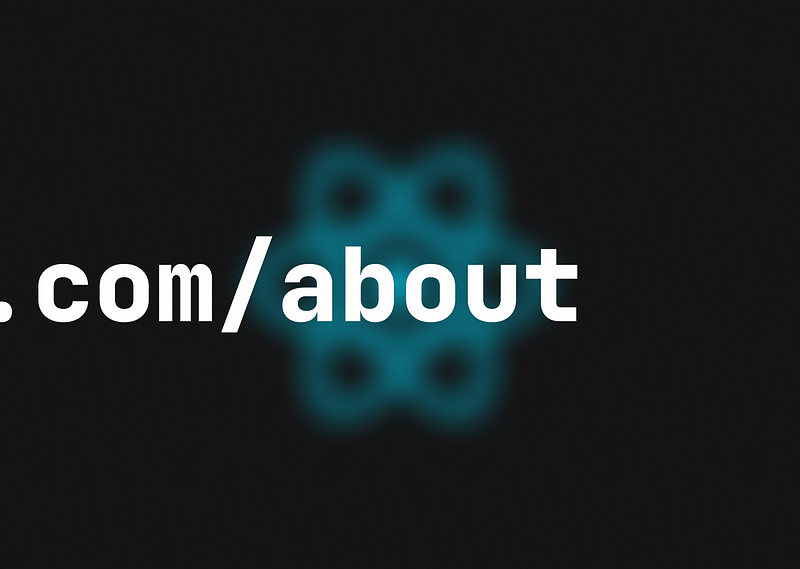
As you may be aware, websites often feature various subpages, each with a unique URL. For instance, consider the following example:
Here, the URL serves as a Uniform Resource Locator (URL), which helps identify resources such as web pages, texts, and images online. This particular URL directs users to the "About" page of awesome.com. In traditional HTML, you would create an <a> tag and set its href attribute to the desired HTML file name. However, in React, we take a different approach by utilizing a powerful library known as React Router.
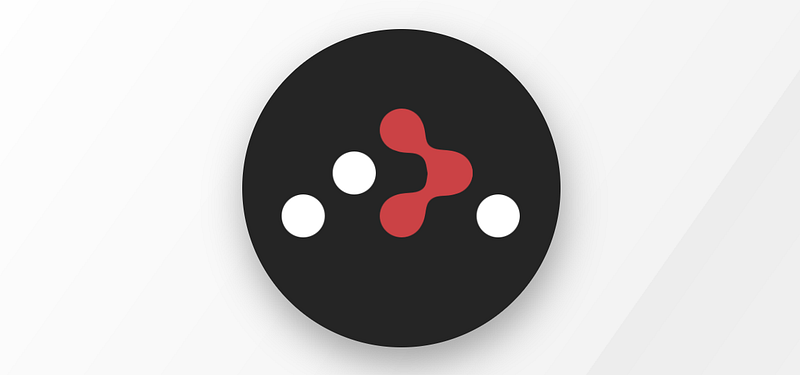
Installation and Setup
In this article, we will focus on React Router version 5. To install it in your project, use either npm or yarn:
npm install [email protected]
or
yarn add [email protected]
Utilizing Routes in Your Application
Imagine being able to specify which component should render for each route in your application. To achieve this, you need to create components for the content of your pages and set up corresponding routes. For example, you could assign the path /about to the <About /> component.
Using React Router, you can define different routes for your page components with wrapper components. First, you will need to implement the <BrowserRouter> component to indicate that you want to use React Router in your browser:
import { BrowserRouter } from "react-router-dom";
const App = () => {
return (
<BrowserRouter>
...
</BrowserRouter>
)
}
Next, you will need a component to encompass all the routes you plan to establish. You can wrap your routes using the <Switch> component:
import { BrowserRouter, Switch } from "react-router-dom";
const App = () => {
return (
<BrowserRouter>
<Switch>
...
</Switch>
</BrowserRouter>
)
}
Defining Unique Paths for Each Component
You're almost there! Now, you just need to specify the unique path for each page component:
import { BrowserRouter, Switch, Route } from "react-router-dom";
const App = () => {
return (
<BrowserRouter>
<Switch>
<Route exact path='/main'>
<Main /></Route>
<Route exact path='/about'>
<About /></Route>
</Switch>
</BrowserRouter>
)
}
With these configurations, your application will be fully functional with distinct routes.
Conclusion
I hope this guide has provided valuable insights into managing routes in React applications. Thank you for taking the time to read this!
Don't forget to subscribe to my email list and follow me on Twitter for more engaging content in the future!
Chapter 2: Video Resources for Further Learning
Explore additional resources to deepen your understanding of routing in React applications.
This video titled "How to Create Pages and Set Up Routes in a React App" provides a comprehensive overview of setting up routing in your React projects.
In this video, "ReactJS Course [8] - React Router Dom | Routes in React," you'll learn more about utilizing React Router for managing routes effectively.